%load_ext autoreload
%autoreload 2
Examples of CORDEX-CMIP6 cmorization#
This notebook should show some examples of how a cmorized CORDEX-CMIP6 dataset could look like.
!ls ../Tables
CORDEX-CMIP6_1hr.json CORDEX-CMIP6_formula_terms.json
CORDEX-CMIP6_6hr.json CORDEX-CMIP6_fx.json
CORDEX-CMIP6_CV.json CORDEX-CMIP6_grids.json
CORDEX-CMIP6_coordinate.json CORDEX-CMIP6_mon.json
CORDEX-CMIP6_day.json CORDEX-CMIP6_remo_example.json
Controlled Vocabulary#
The current CORDEX-CMIP6_CV.json
file contains the controlled vocabulary used by the CMOR3 library for rewriting the model output. Let’s have a look:
import json
import pprint
from IPython.display import JSON
# Opening JSON file
with open("../Tables/CORDEX-CMIP6_CV.json") as json_file:
CV = json.load(json_file)["CV"]
# JSON(CV['required_global_attributes'])
pprint.pprint(CV["required_global_attributes"])
['activity_id',
'contact',
'Conventions',
'creation_date',
'domain_id',
'domain',
'driving_experiment_id',
'driving_experiment',
'driving_institution_id',
'driving_source_id',
'driving_variant_label',
'frequency',
'grid',
'institution',
'institution_id',
'license',
'mip_era',
'product',
'project_id',
'source',
'source_id',
'source_type',
'tracking_id',
'variable_id',
'version_realization']
Cmorization example#
We use the cordex.cmor
module to create an example of a CORDEX-CMIP6 dataset.
Show code cell source
import os
import cordex as cx
import xarray as xr
from cordex import cmor as cmor
table_dir = "../Tables"
cmor.set_options(table_prefix="CORDEX-CMIP6")
def test_cmorizer_fx():
ds = cx.cordex_domain("EUR-11", dummy="topo")
filename = cmor.cmorize_variable(
ds,
"orog",
mapping_table={"orog": {"varname": "topo"}},
cmor_table=os.path.join(table_dir, "CORDEX-CMIP6_fx.json"),
dataset_table=os.path.join(table_dir, "CORDEX-CMIP6_remo_example.json"),
grids_table=os.path.join(table_dir, "CORDEX-CMIP6_grids.json"),
CORDEX_domain="EUR-11",
time_units=None,
allow_units_convert=True,
)
return filename
def test_cmorizer_mon():
ds = cx.tutorial.open_dataset("remo_EUR-11_TEMP2_mon")
filename = cmor.cmorize_variable(
ds,
"tas",
mapping_table={"tas": {"varname": "TEMP2"}},
cmor_table=os.path.join(table_dir, "CORDEX-CMIP6_mon.json"),
dataset_table=os.path.join(table_dir, "CORDEX-CMIP6_remo_example.json"),
grids_table=os.path.join(table_dir, "CORDEX-CMIP6_grids.json"),
CORDEX_domain="EUR-11",
time_units=None,
allow_units_convert=True,
)
return filename
def test_cmorizer_subdaily(table):
ds = cx.tutorial.open_dataset("remo_EUR-11_TEMP2_1hr")
filename = cmor.cmorize_variable(
ds,
"tas",
mapping_table={"tas": {"varname": "TEMP2"}},
cmor_table=os.path.join(table_dir, f"CORDEX-CMIP6_{table}.json"),
dataset_table=os.path.join(table_dir, "CORDEX-CMIP6_remo_example.json"),
grids_table=os.path.join(table_dir, "CORDEX-CMIP6_grids.json"),
CORDEX_domain="EUR-11",
time_units=None,
allow_units_convert=True,
allow_resample=True,
)
return filename
Example from fx
table#
f = test_cmorizer_fx()
Let’s have a look at the filename.
f
'CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/fx/orog/v20250527/orog_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_fx.nc'
!ncdump -h $f
netcdf orog_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_fx {
dimensions:
rlat = 424 ;
rlon = 412 ;
bnds = 2 ;
vertices = 4 ;
variables:
double rlat(rlat) ;
rlat:units = "degrees" ;
rlat:axis = "Y" ;
rlat:long_name = "latitude in rotated pole grid" ;
rlat:standard_name = "grid_latitude" ;
double rlon(rlon) ;
rlon:units = "degrees" ;
rlon:axis = "X" ;
rlon:long_name = "longitude in rotated pole grid" ;
rlon:standard_name = "grid_longitude" ;
int rotated_latitude_longitude ;
rotated_latitude_longitude:grid_mapping_name = "rotated_latitude_longitude" ;
rotated_latitude_longitude:grid_north_pole_latitude = 39.25 ;
rotated_latitude_longitude:grid_north_pole_longitude = -162. ;
rotated_latitude_longitude:north_pole_grid_longitude = 0. ;
double lat(rlat, rlon) ;
lat:standard_name = "latitude" ;
lat:long_name = "latitude" ;
lat:units = "degrees_north" ;
lat:missing_value = 1.e+20 ;
lat:_FillValue = 1.e+20 ;
lat:bounds = "vertices_lat" ;
double lon(rlat, rlon) ;
lon:standard_name = "longitude" ;
lon:long_name = "longitude" ;
lon:units = "degrees_east" ;
lon:missing_value = 1.e+20 ;
lon:_FillValue = 1.e+20 ;
lon:bounds = "vertices_lon" ;
double vertices_lat(rlat, rlon, vertices) ;
vertices_lat:units = "degrees_north" ;
vertices_lat:missing_value = 1.e+20 ;
vertices_lat:_FillValue = 1.e+20 ;
double vertices_lon(rlat, rlon, vertices) ;
vertices_lon:units = "degrees_east" ;
vertices_lon:missing_value = 1.e+20 ;
vertices_lon:_FillValue = 1.e+20 ;
float orog(rlat, rlon) ;
orog:standard_name = "surface_altitude" ;
orog:long_name = "Surface Altitude" ;
orog:units = "m" ;
orog:cell_methods = "area: mean" ;
orog:cell_measures = "area: areacella" ;
orog:history = "2025-05-27T13:41:38Z altered by CMOR: Reordered dimensions, original order: rlon rlat." ;
orog:missing_value = 1.e+20f ;
orog:_FillValue = 1.e+20f ;
orog:grid_mapping = "rotated_latitude_longitude" ;
orog:coordinates = "lat lon" ;
// global attributes:
:Conventions = "CF-1.11" ;
:activity_id = "DD" ;
:contact = "gerics-cordex@hereon.de" ;
:creation_date = "2025-05-27T13:41:38Z" ;
:domain = "Europe" ;
:domain_id = "EUR-12" ;
:driving_experiment = "reanalysis simulation of the recent past" ;
:driving_experiment_id = "evaluation" ;
:driving_institution_id = "ECMWF" ;
:driving_source = "ECMWF Reanalysis v5" ;
:driving_source_id = "ERA5" ;
:driving_variant_label = "r1i1p1f1" ;
:experiment_id = "evaluation" ;
:external_variables = "areacella" ;
:frequency = "fx" ;
:grid = "Rotated-pole latitude-longitude with 0.11 degree grid spacing" ;
:history = "2025-05-27T13:41:38Z ;rewrote data to be consistent with CORDEX-CMIP6 for variable orog found in table fx." ;
:institution = "Climate Service Center Germany, Helmholtz Centre hereon GmbH, Hamburg, Germany" ;
:institution_id = "GERICS" ;
:label = "REMO2020 v2.2" ;
:mip_era = "CMIP6" ;
:product = "model-output" ;
:project_id = "CORDEX-CMIP6" ;
:references = "https://www.remo-rcm.de" ;
:run_variant = "1st realization" ;
:source = "Regional Climate Model REMO, version 2.2, hydrostatic configuration with MACv2 aerosol forcing and Fresh-water Lake model (FLake) (2023)" ;
:source_id = "REMO2020-2-2" ;
:source_type = "ARCM" ;
:table_id = "fx" ;
:table_info = "Creation Date:(27 May 2025) MD5:083889e5c2e7ef27dbc26a4007e20353" ;
:title = "REMO2020-2-2 output prepared for CMIP6" ;
:tracking_id = "hdl:21.14103/b2a43fe4-0055-4205-acb3-d16e5ac69c1b" ;
:variable_id = "orog" ;
:version_realization = "v1-r1" ;
:license = "https://cordex.org/data-access/cordex-cmip6-data/cordex-cmip6-terms-of-use" ;
:cmor_version = "3.9.0" ;
}
The xarray dataset representation allows to explore the dataset interactively.
ds = xr.open_dataset(f)
ds
<xarray.Dataset> Size: 15MB Dimensions: (rlat: 424, rlon: 412, vertices: 4) Coordinates: * rlat (rlat) float64 3kB -28.38 -28.27 ... 18.05 18.16 * rlon (rlon) float64 3kB -23.38 -23.27 ... 21.73 21.84 lat (rlat, rlon) float64 1MB ... lon (rlat, rlon) float64 1MB ... Dimensions without coordinates: vertices Data variables: rotated_latitude_longitude int32 4B ... vertices_lat (rlat, rlon, vertices) float64 6MB ... vertices_lon (rlat, rlon, vertices) float64 6MB ... orog (rlat, rlon) float32 699kB ... Attributes: (12/36) Conventions: CF-1.11 activity_id: DD contact: gerics-cordex@hereon.de creation_date: 2025-05-27T13:41:38Z domain: Europe domain_id: EUR-12 ... ... title: REMO2020-2-2 output prepared for CMIP6 tracking_id: hdl:21.14103/b2a43fe4-0055-4205-acb3-d16e5ac69c1b variable_id: orog version_realization: v1-r1 license: https://cordex.org/data-access/cordex-cmip6-data... cmor_version: 3.9.0
ds.orog.plot()
<matplotlib.collections.QuadMesh at 0x7faecb797ee0>
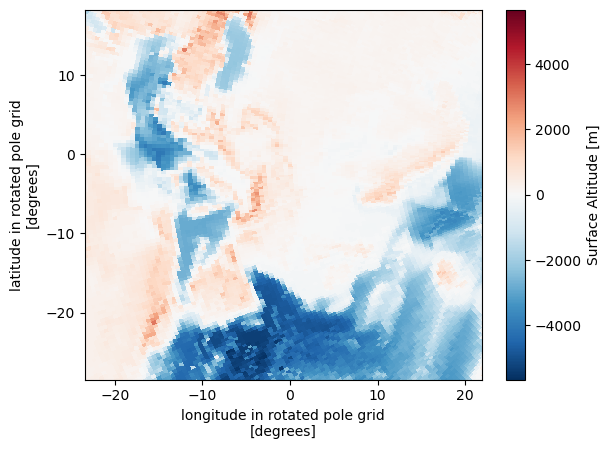
!cdo verifygrid $f
cdo verifygrid: Grid consists of 174688 (412x424) cells (type: curvilinear), of which
cdo verifygrid: 174688 cells have 4 vertices
cdo verifygrid: lon : -44.59386 to 64.96438 degrees
cdo verifygrid: lat : 21.98783 to 72.585 degrees
cdo verifygrid: Processed 1 variable [0.11s 664MB]
!cfchecks $f
CHECKING NetCDF FILE: CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/fx/orog/v20250527/orog_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_fx.nc
=====================
Using CF Checker Version 4.1.0
Checking against CF Version CF-1.8
Using Standard Name Table Version 91 (2025-05-14T10:06:07Z)
Using Area Type Table Version 13 (20 March 2025)
Using Standardized Region Name Table Version 5 (12 November 2024)
ERROR: (2.6.1): This netCDF file does not appear to contain CF Convention data.
WARN: (7.1): Boundary var vertices_lat should not have attribute units
WARN: (7.1): Boundary var vertices_lon should not have attribute units
------------------
Checking variable: rlat
------------------
------------------
Checking variable: rlon
------------------
------------------
Checking variable: rotated_latitude_longitude
------------------
------------------
Checking variable: lat
------------------
------------------
Checking variable: lon
------------------
------------------
Checking variable: vertices_lat
------------------
WARN: (7.1): Boundary Variable vertices_lat should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lat should not have missing_value attribute
------------------
Checking variable: vertices_lon
------------------
WARN: (7.1): Boundary Variable vertices_lon should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lon should not have missing_value attribute
------------------
Checking variable: orog
------------------
INFO: attribute history is being used in a non-standard way
ERRORS detected: 1
WARNINGS given: 6
INFORMATION messages: 1
!compliance-checker --test=cf:1.7 $f
Running Compliance Checker on the datasets from: ['CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/fx/orog/v20250527/orog_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_fx.nc']
--------------------------------------------------------------------------------
IOOS Compliance Checker Report
Version 5.1.1
Report generated 2025-05-27T13:41:41Z
cf:1.7
http://cfconventions.org/Data/cf-conventions/cf-conventions-1.7/cf-conventions.html
--------------------------------------------------------------------------------
Corrective Actions
orog_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_fx.nc has 2 potential issues
Warnings
--------------------------------------------------------------------------------
§2.6 Attributes
* §2.6.1 Conventions global attribute does not contain "CF-1.7"
§7.1 Cell Boundaries
* The Boundary variables 'vertices_lat' should not have the attributes: '['units', 'missing_value', '_FillValue']'
* The Boundary variables 'vertices_lon' should not have the attributes: '['units', 'missing_value', '_FillValue']'
WARNING: The following exceptions occurred during the cf:1.7 checker (possibly indicate compliance checker issues):
cf:1.7.check_cell_boundaries_interval: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
Example from mon
table#
f = test_cmorizer_mon()
f
Downloading data from 'https://github.com/euro-cordex/py-cordex-data/raw/main/remo_EUR-11_TEMP2_mon.nc' to file '/home/runner/.cache/py-cordex_tutorial_data/6d6ccde2e368653ce62a71c80e75f890-remo_EUR-11_TEMP2_mon.nc'.
SHA256 hash of downloaded file: a585a11475492241a7a52963bbdf323af185163166e52924f1a04b4ff91b3ae6
Use this value as the 'known_hash' argument of 'pooch.retrieve' to ensure that the file hasn't changed if it is downloaded again in the future.
/tmp/ipykernel_3615/3805428128.py:30: DeprecationWarning: 'CORDEX_domain' keyword is deprecated, please use the 'domain_id' keyword instead
filename = cmor.cmorize_variable(
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:633: UserWarning: adding time bounds
warn("adding time bounds")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:424: UserWarning: time units are set to default: days since 1950-01-01T00:00:00
warn(f"time units are set to default: {u}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/utils.py:334: UserWarning: writing temporary table to /tmp/tmp8_k0ys2k
warn(f"writing temporary table to {filename}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
'CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/mon/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_mon_200001-200012.nc'
ds = xr.open_dataset(f)
ds
<xarray.Dataset> Size: 22MB Dimensions: (time: 12, bnds: 2, rlat: 424, rlon: 412, vertices: 4) Coordinates: * time (time) datetime64[ns] 96B 2000-01-16T12:00:00... * rlat (rlat) float64 3kB -28.38 -28.27 ... 18.05 18.16 * rlon (rlon) float64 3kB -23.38 -23.27 ... 21.73 21.84 lat (rlat, rlon) float64 1MB ... lon (rlat, rlon) float64 1MB ... height float64 8B ... Dimensions without coordinates: bnds, vertices Data variables: time_bnds (time, bnds) datetime64[ns] 192B ... rotated_latitude_longitude int32 4B ... vertices_lat (rlat, rlon, vertices) float64 6MB ... vertices_lon (rlat, rlon, vertices) float64 6MB ... tas (time, rlat, rlon) float32 8MB ... Attributes: (12/36) Conventions: CF-1.11 activity_id: DD contact: gerics-cordex@hereon.de creation_date: 2025-05-27T13:41:42Z domain: Europe domain_id: EUR-12 ... ... title: REMO2020-2-2 output prepared for CMIP6 tracking_id: hdl:21.14103/985c05c0-9e65-4860-baef-ea18629b6fe3 variable_id: tas version_realization: v1-r1 license: https://cordex.org/data-access/cordex-cmip6-data... cmor_version: 3.9.0
ds.cf
Coordinates:
CF Axes: * X: ['rlon']
* Y: ['rlat']
Z: ['height']
* T: ['time']
CF Coordinates: longitude: ['lon']
latitude: ['lat']
vertical: ['height']
* time: ['time']
Cell Measures: area, volume: n/a
Standard Names: * grid_latitude: ['rlat']
* grid_longitude: ['rlon']
height: ['height']
latitude: ['lat']
longitude: ['lon']
* time: ['time']
Bounds: n/a
Grid Mappings: n/a
Data Variables:
Cell Measures: area, volume: n/a
Standard Names: air_temperature: ['tas']
Bounds: T: ['time_bnds']
lat: ['vertices_lat']
latitude: ['vertices_lat']
lon: ['vertices_lon']
longitude: ['vertices_lon']
time: ['time_bnds']
Grid Mappings: rotated_latitude_longitude: ['rotated_latitude_longitude']
ds.tas.plot(col="time", col_wrap=4)
<xarray.plot.facetgrid.FacetGrid at 0x7faecb6aea60>
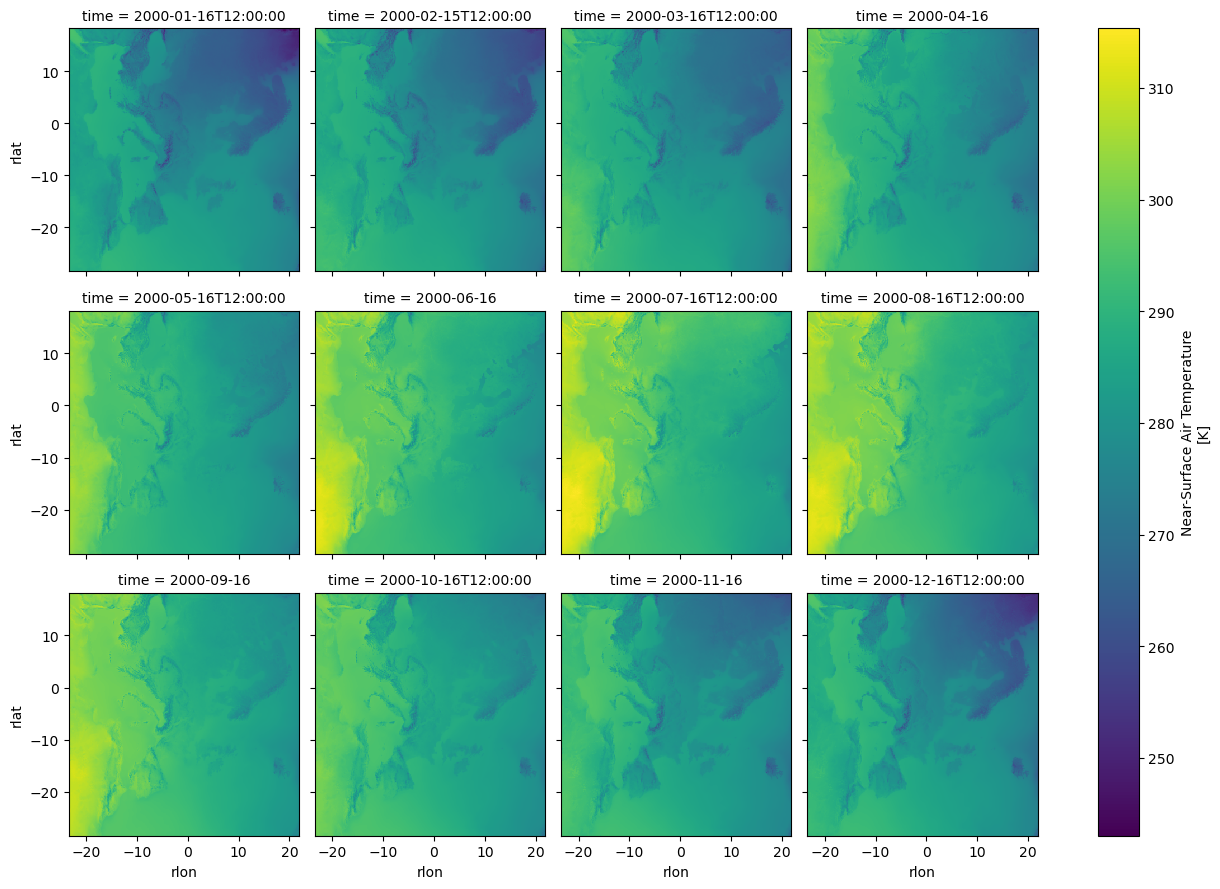
!ncdump -h $f
netcdf tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_mon_200001-200012 {
dimensions:
time = UNLIMITED ; // (12 currently)
rlat = 424 ;
rlon = 412 ;
bnds = 2 ;
vertices = 4 ;
variables:
double time(time) ;
time:bounds = "time_bnds" ;
time:units = "days since 1950-01-01T00:00:00" ;
time:calendar = "proleptic_gregorian" ;
time:axis = "T" ;
time:long_name = "time" ;
time:standard_name = "time" ;
double time_bnds(time, bnds) ;
double rlat(rlat) ;
rlat:units = "degrees" ;
rlat:axis = "Y" ;
rlat:long_name = "latitude in rotated pole grid" ;
rlat:standard_name = "grid_latitude" ;
double rlon(rlon) ;
rlon:units = "degrees" ;
rlon:axis = "X" ;
rlon:long_name = "longitude in rotated pole grid" ;
rlon:standard_name = "grid_longitude" ;
int rotated_latitude_longitude ;
rotated_latitude_longitude:grid_mapping_name = "rotated_latitude_longitude" ;
rotated_latitude_longitude:grid_north_pole_latitude = 39.25 ;
rotated_latitude_longitude:grid_north_pole_longitude = -162. ;
rotated_latitude_longitude:north_pole_grid_longitude = 0. ;
double lat(rlat, rlon) ;
lat:standard_name = "latitude" ;
lat:long_name = "latitude" ;
lat:units = "degrees_north" ;
lat:missing_value = 1.e+20 ;
lat:_FillValue = 1.e+20 ;
lat:bounds = "vertices_lat" ;
double lon(rlat, rlon) ;
lon:standard_name = "longitude" ;
lon:long_name = "longitude" ;
lon:units = "degrees_east" ;
lon:missing_value = 1.e+20 ;
lon:_FillValue = 1.e+20 ;
lon:bounds = "vertices_lon" ;
double vertices_lat(rlat, rlon, vertices) ;
vertices_lat:units = "degrees_north" ;
vertices_lat:missing_value = 1.e+20 ;
vertices_lat:_FillValue = 1.e+20 ;
double vertices_lon(rlat, rlon, vertices) ;
vertices_lon:units = "degrees_east" ;
vertices_lon:missing_value = 1.e+20 ;
vertices_lon:_FillValue = 1.e+20 ;
double height ;
height:units = "m" ;
height:axis = "Z" ;
height:positive = "up" ;
height:long_name = "height" ;
height:standard_name = "height" ;
float tas(time, rlat, rlon) ;
tas:standard_name = "air_temperature" ;
tas:long_name = "Near-Surface Air Temperature" ;
tas:units = "K" ;
tas:cell_methods = "area: time: mean" ;
tas:cell_measures = "area: areacella" ;
tas:history = "2025-05-27T13:41:42Z altered by CMOR: Treated scalar dimension: \'height\'. 2025-05-27T13:41:42Z altered by CMOR: Reordered dimensions, original order: time rlon rlat." ;
tas:coordinates = "height lat lon" ;
tas:missing_value = 1.e+20f ;
tas:_FillValue = 1.e+20f ;
tas:grid_mapping = "rotated_latitude_longitude" ;
// global attributes:
:Conventions = "CF-1.11" ;
:activity_id = "DD" ;
:contact = "gerics-cordex@hereon.de" ;
:creation_date = "2025-05-27T13:41:42Z" ;
:domain = "Europe" ;
:domain_id = "EUR-12" ;
:driving_experiment = "reanalysis simulation of the recent past" ;
:driving_experiment_id = "evaluation" ;
:driving_institution_id = "ECMWF" ;
:driving_source = "ECMWF Reanalysis v5" ;
:driving_source_id = "ERA5" ;
:driving_variant_label = "r1i1p1f1" ;
:experiment_id = "evaluation" ;
:external_variables = "areacella" ;
:frequency = "mon" ;
:grid = "Rotated-pole latitude-longitude with 0.11 degree grid spacing" ;
:history = "2025-05-27T13:41:42Z ;rewrote data to be consistent with CORDEX-CMIP6 for variable tas found in table mon." ;
:institution = "Climate Service Center Germany, Helmholtz Centre hereon GmbH, Hamburg, Germany" ;
:institution_id = "GERICS" ;
:label = "REMO2020 v2.2" ;
:mip_era = "CMIP6" ;
:product = "model-output" ;
:project_id = "CORDEX-CMIP6" ;
:references = "https://www.remo-rcm.de" ;
:run_variant = "1st realization" ;
:source = "Regional Climate Model REMO, version 2.2, hydrostatic configuration with MACv2 aerosol forcing and Fresh-water Lake model (FLake) (2023)" ;
:source_id = "REMO2020-2-2" ;
:source_type = "ARCM" ;
:table_id = "mon" ;
:table_info = "Creation Date:(27 May 2025) MD5:083889e5c2e7ef27dbc26a4007e20353" ;
:title = "REMO2020-2-2 output prepared for CMIP6" ;
:tracking_id = "hdl:21.14103/985c05c0-9e65-4860-baef-ea18629b6fe3" ;
:variable_id = "tas" ;
:version_realization = "v1-r1" ;
:license = "https://cordex.org/data-access/cordex-cmip6-data/cordex-cmip6-terms-of-use" ;
:cmor_version = "3.9.0" ;
}
!cfchecks $f
CHECKING NetCDF FILE: CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/mon/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_mon_200001-200012.nc
=====================
Using CF Checker Version 4.1.0
Checking against CF Version CF-1.8
Using Standard Name Table Version 91 (2025-05-14T10:06:07Z)
Using Area Type Table Version 13 (20 March 2025)
Using Standardized Region Name Table Version 5 (12 November 2024)
ERROR: (2.6.1): This netCDF file does not appear to contain CF Convention data.
WARN: (7.1): Boundary var vertices_lat should not have attribute units
WARN: (7.1): Boundary var vertices_lon should not have attribute units
------------------
Checking variable: time
------------------
------------------
Checking variable: time_bnds
------------------
------------------
Checking variable: rlat
------------------
------------------
Checking variable: rlon
------------------
------------------
Checking variable: rotated_latitude_longitude
------------------
------------------
Checking variable: lat
------------------
------------------
Checking variable: lon
------------------
------------------
Checking variable: vertices_lat
------------------
WARN: (7.1): Boundary Variable vertices_lat should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lat should not have missing_value attribute
------------------
Checking variable: vertices_lon
------------------
WARN: (7.1): Boundary Variable vertices_lon should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lon should not have missing_value attribute
------------------
Checking variable: height
------------------
------------------
Checking variable: tas
------------------
INFO: attribute history is being used in a non-standard way
ERRORS detected: 1
WARNINGS given: 6
INFORMATION messages: 1
!compliance-checker --test=cf:1.7 $f
Running Compliance Checker on the datasets from: ['CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/mon/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_mon_200001-200012.nc']
--------------------------------------------------------------------------------
IOOS Compliance Checker Report
Version 5.1.1
Report generated 2025-05-27T13:41:48Z
cf:1.7
http://cfconventions.org/Data/cf-conventions/cf-conventions-1.7/cf-conventions.html
--------------------------------------------------------------------------------
Corrective Actions
tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_mon_200001-200012.nc has 2 potential issues
Warnings
--------------------------------------------------------------------------------
§2.6 Attributes
* §2.6.1 Conventions global attribute does not contain "CF-1.7"
§7.1 Cell Boundaries
* The Boundary variables 'vertices_lat' should not have the attributes: '['units', 'missing_value', '_FillValue']'
* The Boundary variables 'vertices_lon' should not have the attributes: '['units', 'missing_value', '_FillValue']'
Example from daily and subdaily tables#
f = test_cmorizer_subdaily("1hr")
f
Downloading data from 'https://github.com/euro-cordex/py-cordex-data/raw/main/remo_EUR-11_TEMP2_1hr.nc' to file '/home/runner/.cache/py-cordex_tutorial_data/df1ad3551d1e9e1f102d1a1536d5212c-remo_EUR-11_TEMP2_1hr.nc'.
SHA256 hash of downloaded file: 0ddd4c71be010a5656ed624aa6bfd3c3fa6238d8c61c9f39b1494c4188f060a8
Use this value as the 'known_hash' argument of 'pooch.retrieve' to ensure that the file hasn't changed if it is downloaded again in the future.
/tmp/ipykernel_3615/3805428128.py:46: DeprecationWarning: 'CORDEX_domain' keyword is deprecated, please use the 'domain_id' keyword instead
filename = cmor.cmorize_variable(
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:424: UserWarning: time units are set to default: days since 1950-01-01T00:00:00
warn(f"time units are set to default: {u}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/utils.py:334: UserWarning: writing temporary table to /tmp/tmprnkiwvfd
warn(f"writing temporary table to {filename}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
'CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/1hr/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_1hr_200001010000-200001030000.nc'
ds = xr.open_dataset(f)
ds
<xarray.Dataset> Size: 48MB Dimensions: (time: 49, rlat: 424, rlon: 412, vertices: 4) Coordinates: * time (time) datetime64[ns] 392B 2000-01-01 ... 200... * rlat (rlat) float64 3kB -28.38 -28.27 ... 18.05 18.16 * rlon (rlon) float64 3kB -23.38 -23.27 ... 21.73 21.84 lat (rlat, rlon) float64 1MB ... lon (rlat, rlon) float64 1MB ... height float64 8B ... Dimensions without coordinates: vertices Data variables: rotated_latitude_longitude int32 4B ... vertices_lat (rlat, rlon, vertices) float64 6MB ... vertices_lon (rlat, rlon, vertices) float64 6MB ... tas (time, rlat, rlon) float32 34MB ... Attributes: (12/36) Conventions: CF-1.11 activity_id: DD contact: gerics-cordex@hereon.de creation_date: 2025-05-27T13:41:49Z domain: Europe domain_id: EUR-12 ... ... title: REMO2020-2-2 output prepared for CMIP6 tracking_id: hdl:21.14103/9b8a2987-9796-4759-9031-ee477367987f variable_id: tas version_realization: v1-r1 license: https://cordex.org/data-access/cordex-cmip6-data... cmor_version: 3.9.0
!ncdump -h $f
netcdf tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_1hr_200001010000-200001030000 {
dimensions:
time = UNLIMITED ; // (49 currently)
rlat = 424 ;
rlon = 412 ;
bnds = 2 ;
vertices = 4 ;
variables:
double time(time) ;
time:units = "days since 1950-01-01T00:00:00" ;
time:calendar = "proleptic_gregorian" ;
time:axis = "T" ;
time:long_name = "time" ;
time:standard_name = "time" ;
double rlat(rlat) ;
rlat:units = "degrees" ;
rlat:axis = "Y" ;
rlat:long_name = "latitude in rotated pole grid" ;
rlat:standard_name = "grid_latitude" ;
double rlon(rlon) ;
rlon:units = "degrees" ;
rlon:axis = "X" ;
rlon:long_name = "longitude in rotated pole grid" ;
rlon:standard_name = "grid_longitude" ;
int rotated_latitude_longitude ;
rotated_latitude_longitude:grid_mapping_name = "rotated_latitude_longitude" ;
rotated_latitude_longitude:grid_north_pole_latitude = 39.25 ;
rotated_latitude_longitude:grid_north_pole_longitude = -162. ;
rotated_latitude_longitude:north_pole_grid_longitude = 0. ;
double lat(rlat, rlon) ;
lat:standard_name = "latitude" ;
lat:long_name = "latitude" ;
lat:units = "degrees_north" ;
lat:missing_value = 1.e+20 ;
lat:_FillValue = 1.e+20 ;
lat:bounds = "vertices_lat" ;
double lon(rlat, rlon) ;
lon:standard_name = "longitude" ;
lon:long_name = "longitude" ;
lon:units = "degrees_east" ;
lon:missing_value = 1.e+20 ;
lon:_FillValue = 1.e+20 ;
lon:bounds = "vertices_lon" ;
double vertices_lat(rlat, rlon, vertices) ;
vertices_lat:units = "degrees_north" ;
vertices_lat:missing_value = 1.e+20 ;
vertices_lat:_FillValue = 1.e+20 ;
double vertices_lon(rlat, rlon, vertices) ;
vertices_lon:units = "degrees_east" ;
vertices_lon:missing_value = 1.e+20 ;
vertices_lon:_FillValue = 1.e+20 ;
double height ;
height:units = "m" ;
height:axis = "Z" ;
height:positive = "up" ;
height:long_name = "height" ;
height:standard_name = "height" ;
float tas(time, rlat, rlon) ;
tas:standard_name = "air_temperature" ;
tas:long_name = "Near-Surface Air Temperature" ;
tas:units = "K" ;
tas:cell_methods = "area: mean time: point" ;
tas:cell_measures = "area: areacella" ;
tas:history = "2025-05-27T13:41:49Z altered by CMOR: Treated scalar dimension: \'height\'. 2025-05-27T13:41:49Z altered by CMOR: Reordered dimensions, original order: time rlon rlat." ;
tas:coordinates = "height lat lon" ;
tas:missing_value = 1.e+20f ;
tas:_FillValue = 1.e+20f ;
tas:grid_mapping = "rotated_latitude_longitude" ;
// global attributes:
:Conventions = "CF-1.11" ;
:activity_id = "DD" ;
:contact = "gerics-cordex@hereon.de" ;
:creation_date = "2025-05-27T13:41:49Z" ;
:domain = "Europe" ;
:domain_id = "EUR-12" ;
:driving_experiment = "reanalysis simulation of the recent past" ;
:driving_experiment_id = "evaluation" ;
:driving_institution_id = "ECMWF" ;
:driving_source = "ECMWF Reanalysis v5" ;
:driving_source_id = "ERA5" ;
:driving_variant_label = "r1i1p1f1" ;
:experiment_id = "evaluation" ;
:external_variables = "areacella" ;
:frequency = "1hr" ;
:grid = "Rotated-pole latitude-longitude with 0.11 degree grid spacing" ;
:history = "2025-05-27T13:41:49Z ;rewrote data to be consistent with CORDEX-CMIP6 for variable tas found in table 1hr." ;
:institution = "Climate Service Center Germany, Helmholtz Centre hereon GmbH, Hamburg, Germany" ;
:institution_id = "GERICS" ;
:label = "REMO2020 v2.2" ;
:mip_era = "CMIP6" ;
:product = "model-output" ;
:project_id = "CORDEX-CMIP6" ;
:references = "https://www.remo-rcm.de" ;
:run_variant = "1st realization" ;
:source = "Regional Climate Model REMO, version 2.2, hydrostatic configuration with MACv2 aerosol forcing and Fresh-water Lake model (FLake) (2023)" ;
:source_id = "REMO2020-2-2" ;
:source_type = "ARCM" ;
:table_id = "1hr" ;
:table_info = "Creation Date:(27 May 2025) MD5:083889e5c2e7ef27dbc26a4007e20353" ;
:title = "REMO2020-2-2 output prepared for CMIP6" ;
:tracking_id = "hdl:21.14103/9b8a2987-9796-4759-9031-ee477367987f" ;
:variable_id = "tas" ;
:version_realization = "v1-r1" ;
:license = "https://cordex.org/data-access/cordex-cmip6-data/cordex-cmip6-terms-of-use" ;
:cmor_version = "3.9.0" ;
}
!cfchecks $f
CHECKING NetCDF FILE: CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/1hr/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_1hr_200001010000-200001030000.nc
=====================
Using CF Checker Version 4.1.0
Checking against CF Version CF-1.8
Using Standard Name Table Version 91 (2025-05-14T10:06:07Z)
Using Area Type Table Version 13 (20 March 2025)
Using Standardized Region Name Table Version 5 (12 November 2024)
ERROR: (2.6.1): This netCDF file does not appear to contain CF Convention data.
WARN: (7.1): Boundary var vertices_lat should not have attribute units
WARN: (7.1): Boundary var vertices_lon should not have attribute units
------------------
Checking variable: time
------------------
------------------
Checking variable: rlat
------------------
------------------
Checking variable: rlon
------------------
------------------
Checking variable: rotated_latitude_longitude
------------------
------------------
Checking variable: lat
------------------
------------------
Checking variable: lon
------------------
------------------
Checking variable: vertices_lat
------------------
WARN: (7.1): Boundary Variable vertices_lat should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lat should not have missing_value attribute
------------------
Checking variable: vertices_lon
------------------
WARN: (7.1): Boundary Variable vertices_lon should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lon should not have missing_value attribute
------------------
Checking variable: height
------------------
------------------
Checking variable: tas
------------------
INFO: attribute history is being used in a non-standard way
ERRORS detected: 1
WARNINGS given: 6
INFORMATION messages: 1
!compliance-checker --test=cf:1.7 $f
Running Compliance Checker on the datasets from: ['CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/1hr/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_1hr_200001010000-200001030000.nc']
--------------------------------------------------------------------------------
IOOS Compliance Checker Report
Version 5.1.1
Report generated 2025-05-27T13:41:52Z
cf:1.7
http://cfconventions.org/Data/cf-conventions/cf-conventions-1.7/cf-conventions.html
--------------------------------------------------------------------------------
Corrective Actions
tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_1hr_200001010000-200001030000.nc has 2 potential issues
Warnings
--------------------------------------------------------------------------------
§2.6 Attributes
* §2.6.1 Conventions global attribute does not contain "CF-1.7"
§7.1 Cell Boundaries
* The Boundary variables 'vertices_lat' should not have the attributes: '['units', 'missing_value', '_FillValue']'
* The Boundary variables 'vertices_lon' should not have the attributes: '['units', 'missing_value', '_FillValue']'
WARNING: The following exceptions occurred during the cf:1.7 checker (possibly indicate compliance checker issues):
cf:1.7.check_cell_boundaries_interval: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
# cordex.cmor will automatically resample hourly to other frequencies
# depending on the frequency in the cmor table and the frequency in the
# input dataset
f = test_cmorizer_subdaily("day")
f
/tmp/ipykernel_3615/3805428128.py:46: DeprecationWarning: 'CORDEX_domain' keyword is deprecated, please use the 'domain_id' keyword instead
filename = cmor.cmorize_variable(
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:515: UserWarning: resampling input data from H to D
warn(f"resampling input data from {input_freq} to {pd_freq}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:633: UserWarning: adding time bounds
warn("adding time bounds")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/cmor.py:424: UserWarning: time units are set to default: days since 1950-01-01T00:00:00
warn(f"time units are set to default: {u}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cordex/cmor/utils.py:334: UserWarning: writing temporary table to /tmp/tmpp80bgua7
warn(f"writing temporary table to {filename}")
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:701: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
unused_keys = set(attribute.keys()) - set(inverted)
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:702: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
for key, value in attribute.items():
/home/runner/micromamba/envs/cordex-examples/lib/python3.9/site-packages/cf_xarray/accessor.py:710: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
newmap.update({key: attribute[key] for key in unused_keys})
'CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/day/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_day_19991231-20000102.nc'
ds = xr.open_dataset(f)
ds
<xarray.Dataset> Size: 16MB Dimensions: (time: 3, bnds: 2, rlat: 424, rlon: 412, vertices: 4) Coordinates: * time (time) datetime64[ns] 24B 1999-12-31T12:00:00... * rlat (rlat) float64 3kB -28.38 -28.27 ... 18.05 18.16 * rlon (rlon) float64 3kB -23.38 -23.27 ... 21.73 21.84 lat (rlat, rlon) float64 1MB ... lon (rlat, rlon) float64 1MB ... height float64 8B ... Dimensions without coordinates: bnds, vertices Data variables: time_bnds (time, bnds) datetime64[ns] 48B ... rotated_latitude_longitude int32 4B ... vertices_lat (rlat, rlon, vertices) float64 6MB ... vertices_lon (rlat, rlon, vertices) float64 6MB ... tas (time, rlat, rlon) float32 2MB ... Attributes: (12/36) Conventions: CF-1.11 activity_id: DD contact: gerics-cordex@hereon.de creation_date: 2025-05-27T13:41:52Z domain: Europe domain_id: EUR-12 ... ... title: REMO2020-2-2 output prepared for CMIP6 tracking_id: hdl:21.14103/4afb9d5d-b00a-4188-89b0-00bfd8561331 variable_id: tas version_realization: v1-r1 license: https://cordex.org/data-access/cordex-cmip6-data... cmor_version: 3.9.0
ds.tas.plot(col="time")
<xarray.plot.facetgrid.FacetGrid at 0x7faecb15a370>
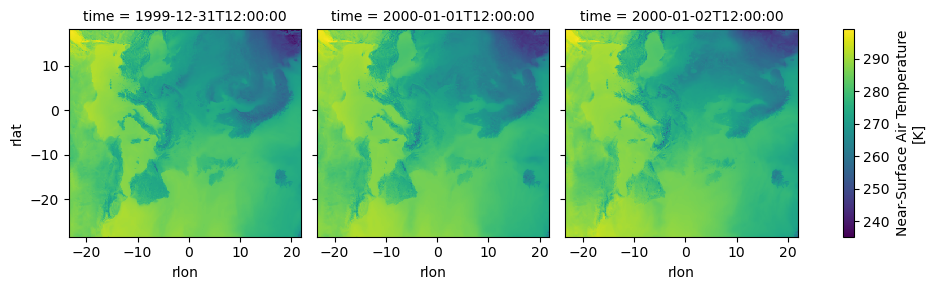
!ncdump -h $f
netcdf tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_day_19991231-20000102 {
dimensions:
time = UNLIMITED ; // (3 currently)
rlat = 424 ;
rlon = 412 ;
bnds = 2 ;
vertices = 4 ;
variables:
double time(time) ;
time:bounds = "time_bnds" ;
time:units = "days since 1950-01-01T00:00:00" ;
time:calendar = "proleptic_gregorian" ;
time:axis = "T" ;
time:long_name = "time" ;
time:standard_name = "time" ;
double time_bnds(time, bnds) ;
double rlat(rlat) ;
rlat:units = "degrees" ;
rlat:axis = "Y" ;
rlat:long_name = "latitude in rotated pole grid" ;
rlat:standard_name = "grid_latitude" ;
double rlon(rlon) ;
rlon:units = "degrees" ;
rlon:axis = "X" ;
rlon:long_name = "longitude in rotated pole grid" ;
rlon:standard_name = "grid_longitude" ;
int rotated_latitude_longitude ;
rotated_latitude_longitude:grid_mapping_name = "rotated_latitude_longitude" ;
rotated_latitude_longitude:grid_north_pole_latitude = 39.25 ;
rotated_latitude_longitude:grid_north_pole_longitude = -162. ;
rotated_latitude_longitude:north_pole_grid_longitude = 0. ;
double lat(rlat, rlon) ;
lat:standard_name = "latitude" ;
lat:long_name = "latitude" ;
lat:units = "degrees_north" ;
lat:missing_value = 1.e+20 ;
lat:_FillValue = 1.e+20 ;
lat:bounds = "vertices_lat" ;
double lon(rlat, rlon) ;
lon:standard_name = "longitude" ;
lon:long_name = "longitude" ;
lon:units = "degrees_east" ;
lon:missing_value = 1.e+20 ;
lon:_FillValue = 1.e+20 ;
lon:bounds = "vertices_lon" ;
double vertices_lat(rlat, rlon, vertices) ;
vertices_lat:units = "degrees_north" ;
vertices_lat:missing_value = 1.e+20 ;
vertices_lat:_FillValue = 1.e+20 ;
double vertices_lon(rlat, rlon, vertices) ;
vertices_lon:units = "degrees_east" ;
vertices_lon:missing_value = 1.e+20 ;
vertices_lon:_FillValue = 1.e+20 ;
double height ;
height:units = "m" ;
height:axis = "Z" ;
height:positive = "up" ;
height:long_name = "height" ;
height:standard_name = "height" ;
float tas(time, rlat, rlon) ;
tas:standard_name = "air_temperature" ;
tas:long_name = "Near-Surface Air Temperature" ;
tas:units = "K" ;
tas:cell_methods = "area: time: mean" ;
tas:cell_measures = "area: areacella" ;
tas:history = "2025-05-27T13:41:52Z altered by CMOR: Treated scalar dimension: \'height\'. 2025-05-27T13:41:52Z altered by CMOR: Reordered dimensions, original order: time rlon rlat." ;
tas:coordinates = "height lat lon" ;
tas:missing_value = 1.e+20f ;
tas:_FillValue = 1.e+20f ;
tas:grid_mapping = "rotated_latitude_longitude" ;
// global attributes:
:Conventions = "CF-1.11" ;
:activity_id = "DD" ;
:contact = "gerics-cordex@hereon.de" ;
:creation_date = "2025-05-27T13:41:52Z" ;
:domain = "Europe" ;
:domain_id = "EUR-12" ;
:driving_experiment = "reanalysis simulation of the recent past" ;
:driving_experiment_id = "evaluation" ;
:driving_institution_id = "ECMWF" ;
:driving_source = "ECMWF Reanalysis v5" ;
:driving_source_id = "ERA5" ;
:driving_variant_label = "r1i1p1f1" ;
:experiment_id = "evaluation" ;
:external_variables = "areacella" ;
:frequency = "day" ;
:grid = "Rotated-pole latitude-longitude with 0.11 degree grid spacing" ;
:history = "2025-05-27T13:41:52Z ;rewrote data to be consistent with CORDEX-CMIP6 for variable tas found in table day." ;
:institution = "Climate Service Center Germany, Helmholtz Centre hereon GmbH, Hamburg, Germany" ;
:institution_id = "GERICS" ;
:label = "REMO2020 v2.2" ;
:mip_era = "CMIP6" ;
:product = "model-output" ;
:project_id = "CORDEX-CMIP6" ;
:references = "https://www.remo-rcm.de" ;
:run_variant = "1st realization" ;
:source = "Regional Climate Model REMO, version 2.2, hydrostatic configuration with MACv2 aerosol forcing and Fresh-water Lake model (FLake) (2023)" ;
:source_id = "REMO2020-2-2" ;
:source_type = "ARCM" ;
:table_id = "day" ;
:table_info = "Creation Date:(27 May 2025) MD5:083889e5c2e7ef27dbc26a4007e20353" ;
:title = "REMO2020-2-2 output prepared for CMIP6" ;
:tracking_id = "hdl:21.14103/4afb9d5d-b00a-4188-89b0-00bfd8561331" ;
:variable_id = "tas" ;
:version_realization = "v1-r1" ;
:license = "https://cordex.org/data-access/cordex-cmip6-data/cordex-cmip6-terms-of-use" ;
:cmor_version = "3.9.0" ;
}
!cfchecks $f
CHECKING NetCDF FILE: CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/day/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_day_19991231-20000102.nc
=====================
Using CF Checker Version 4.1.0
Checking against CF Version CF-1.8
Using Standard Name Table Version 91 (2025-05-14T10:06:07Z)
Using Area Type Table Version 13 (20 March 2025)
Using Standardized Region Name Table Version 5 (12 November 2024)
ERROR: (2.6.1): This netCDF file does not appear to contain CF Convention data.
WARN: (7.1): Boundary var vertices_lat should not have attribute units
WARN: (7.1): Boundary var vertices_lon should not have attribute units
------------------
Checking variable: time
------------------
------------------
Checking variable: time_bnds
------------------
------------------
Checking variable: rlat
------------------
------------------
Checking variable: rlon
------------------
------------------
Checking variable: rotated_latitude_longitude
------------------
------------------
Checking variable: lat
------------------
------------------
Checking variable: lon
------------------
------------------
Checking variable: vertices_lat
------------------
WARN: (7.1): Boundary Variable vertices_lat should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lat should not have missing_value attribute
------------------
Checking variable: vertices_lon
------------------
WARN: (7.1): Boundary Variable vertices_lon should not have _FillValue attribute
WARN: (7.1): Boundary Variable vertices_lon should not have missing_value attribute
------------------
Checking variable: height
------------------
------------------
Checking variable: tas
------------------
INFO: attribute history is being used in a non-standard way
ERRORS detected: 1
WARNINGS given: 6
INFORMATION messages: 1
!compliance-checker --test=cf:1.7 $f
Running Compliance Checker on the datasets from: ['CORDEX/CORDEX-CMIP6/DD/EUR-12/GERICS/ERA5/evaluation/r1i1p1f1/REMO2020-2-2/v1-r1/day/tas/v20250527/tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_day_19991231-20000102.nc']
--------------------------------------------------------------------------------
IOOS Compliance Checker Report
Version 5.1.1
Report generated 2025-05-27T13:41:55Z
cf:1.7
http://cfconventions.org/Data/cf-conventions/cf-conventions-1.7/cf-conventions.html
--------------------------------------------------------------------------------
Corrective Actions
tas_EUR-12_ERA5_evaluation_r1i1p1f1_GERICS_REMO2020-2-2_v1-r1_day_19991231-20000102.nc has 2 potential issues
Warnings
--------------------------------------------------------------------------------
§2.6 Attributes
* §2.6.1 Conventions global attribute does not contain "CF-1.7"
§7.1 Cell Boundaries
* The Boundary variables 'vertices_lat' should not have the attributes: '['units', 'missing_value', '_FillValue']'
* The Boundary variables 'vertices_lon' should not have the attributes: '['units', 'missing_value', '_FillValue']'